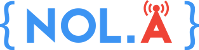 |
SDK
23.9.2
For IoT System Software Development
|
The model of a BLE Characteristic.
More...
#include <BLECharacteristic.hpp>
|
static const uint32_t | PROPERTY_READ = 1<<0 |
|
static const uint32_t | PROPERTY_WRITE = 1<<1 |
|
static const uint32_t | PROPERTY_NOTIFY = 1<<2 |
|
static const uint32_t | PROPERTY_BROADCAST = 1<<3 |
|
static const uint32_t | PROPERTY_INDICATE = 1<<4 |
|
static const uint32_t | PROPERTY_WRITE_NR = 1<<5 |
|
The model of a BLE Characteristic.
A BLE Characteristic is an identified value container that manages a value. It is exposed by a BLE server and can be read and written to by a BLE client.
◆ BLECharacteristic() [1/2]
BLECharacteristic::BLECharacteristic |
( |
const char * |
uuid, |
|
|
uint32_t |
properties = 0 |
|
) |
| |
Construct a characteristic.
- Parameters
-
[in] | uuid | - UUID (const char*) for the characteristic. |
[in] | properties | - Properties for the characteristic. |
◆ BLECharacteristic() [2/2]
BLECharacteristic::BLECharacteristic |
( |
BLEUUID |
uuid, |
|
|
uint32_t |
properties = 0 |
|
) |
| |
Construct a characteristic.
- Parameters
-
[in] | uuid | - UUID for the characteristic. |
[in] | properties | - Properties for the characteristic. |
53 m_handle = NULL_HANDLE;
54 m_properties = (ble_gatt_char_prop_t)0;
55 m_pCallbacks =
nullptr;
◆ addDescriptor()
void BLECharacteristic::addDescriptor |
( |
BLEDescriptor * |
pDescriptor | ) |
|
Associate a descriptor with this characteristic.
- Parameters
-
- Returns
- N/A.
79 void *logCtx __attribute__((unused)) =
nullptr;
80 _debug_print_s(TAG,
">> addDescriptor(): Adding ", pDescriptor->
toString().c_str(), &logCtx);
81 _debug_print_s(TAG,
" to",
toString().c_str(), &logCtx);
83 _debug_print(TAG,
"<< addDescriptor()");
◆ getData()
uint8_t * BLECharacteristic::getData |
( |
| ) |
|
Retrieve the current raw data of the characteristic.
- Returns
- A pointer to storage containing the current characteristic data.
◆ getDescriptorByUUID() [1/2]
Return the BLE Descriptor for the given UUID if associated with this characteristic.
- Parameters
-
[in] | descriptorUUID | The UUID of the descriptor that we wish to retrieve. |
- Returns
- The BLE Descriptor. If no such descriptor is associated with the characteristic, nullptr is returned.
103 return m_descriptorMap.
getByUUID(descriptorUUID);
◆ getDescriptorByUUID() [2/2]
BLEDescriptor * BLECharacteristic::getDescriptorByUUID |
( |
const char * |
descriptorUUID | ) |
|
Return the BLE Descriptor for the given UUID if associated with this characteristic.
- Parameters
-
[in] | descriptorUUID | The UUID of the descriptor that we wish to retrieve. |
- Returns
- The BLE Descriptor. If no such descriptor is associated with the characteristic, nullptr is returned.
◆ getHandle()
uint16_t BLECharacteristic::getHandle |
( |
| ) |
|
Get the handle of the characteristic.
- Returns
- The handle of the characteristic.
◆ getUUID()
BLEUUID BLECharacteristic::getUUID |
( |
| ) |
|
Get the UUID of the characteristic.
- Returns
- The UUID of the characteristic.
◆ getValue()
std::string BLECharacteristic::getValue |
( |
| ) |
|
Retrieve the current value of the characteristic.
- Returns
- A pointer to storage containing the current characteristic value.
◆ indicate()
void BLECharacteristic::indicate |
( |
| ) |
|
Send an indication. An indication is a transmission of up to the first 20 bytes of the characteristic value. An indication will block waiting a positive confirmation from the client.
- Returns
- N/A
165 _debug_print_d(TAG,
">> indicate: length", m_value.
getValue().length());
167 _debug_print(TAG,
"<< indicate");
◆ notify()
void BLECharacteristic::notify |
( |
bool |
is_notification = true | ) |
|
Send a notify. A notification is a transmission of up to the first 20 bytes of the characteristic value. An notification will not block; it is a fire and forget.
- Returns
- N/A.
◆ setBroadcastProperty()
void BLECharacteristic::setBroadcastProperty |
( |
bool |
value | ) |
|
Set the permission to broadcast. A characteristics has properties associated with it which define what it is capable of doing. One of these is the broadcast flag.
- Parameters
-
[in] | value | The flag value of the property. |
- Returns
- N/A
181 m_properties = (ble_gatt_char_prop_t)(m_properties | BLE_GATT_CHAR_PROP_BIT_BROADCAST);
183 m_properties = (ble_gatt_char_prop_t)(m_properties & ~BLE_GATT_CHAR_PROP_BIT_BROADCAST);
◆ setCallbacks()
Set the callback handlers for this characteristic.
- Parameters
-
[in] | pCallbacks | An instance of a callbacks structure used to define any callbacks for the characteristic. |
193 _debug_print_p(TAG,
">> setCallbacks", pCallbacks);
194 m_pCallbacks = pCallbacks;
195 _debug_print(TAG,
"<< setCallbacks");
◆ setIndicateProperty()
void BLECharacteristic::setIndicateProperty |
( |
bool |
value | ) |
|
Set the Indicate property value.
- Parameters
-
[in] | value | Set to true if we are to allow indicate messages. |
225 m_properties = (ble_gatt_char_prop_t)(m_properties | BLE_GATT_CHAR_PROP_BIT_INDICATE);
227 m_properties = (ble_gatt_char_prop_t)(m_properties & ~BLE_GATT_CHAR_PROP_BIT_INDICATE);
◆ setNotifyProperty()
void BLECharacteristic::setNotifyProperty |
( |
bool |
value | ) |
|
Set the Notify property value.
- Parameters
-
[in] | value | Set to true if we are to allow notification messages. |
239 m_properties = (ble_gatt_char_prop_t)(m_properties | BLE_GATT_CHAR_PROP_BIT_NOTIFY);
241 m_properties = (ble_gatt_char_prop_t)(m_properties & ~BLE_GATT_CHAR_PROP_BIT_NOTIFY);
◆ setReadProperty()
void BLECharacteristic::setReadProperty |
( |
bool |
value | ) |
|
Set the Read property value.
- Parameters
-
[in] | value | Set to true if we are to allow reads. |
253 m_properties = (ble_gatt_char_prop_t)(m_properties | BLE_GATT_CHAR_PROP_BIT_READ);
255 m_properties = (ble_gatt_char_prop_t)(m_properties & ~BLE_GATT_CHAR_PROP_BIT_READ);
◆ setValue() [1/2]
void BLECharacteristic::setValue |
( |
std::string |
value | ) |
|
Set the value of the characteristic from string data. We set the value of the characteristic from the bytes contained in the string.
- Parameters
-
[in] | Set | the value of the characteristic. |
- Returns
- N/A.
268 setValue((uint8_t*)(value.data()), value.length());
◆ setValue() [2/2]
void BLECharacteristic::setValue |
( |
uint8_t * |
data, |
|
|
size_t |
size |
|
) |
| |
Set the value of the characteristic.
- Parameters
-
[in] | data | The data to set for the characteristic. |
[in] | length | The length of the data in bytes. |
◆ setWriteNoResponseProperty()
void BLECharacteristic::setWriteNoResponseProperty |
( |
bool |
value | ) |
|
Set the Write No Response property value.
- Parameters
-
[in] | value | Set to true if we are to allow writes with no response. |
318 m_properties = (ble_gatt_char_prop_t)(m_properties | BLE_GATT_CHAR_PROP_BIT_WRITE_NR);
320 m_properties = (ble_gatt_char_prop_t)(m_properties & ~BLE_GATT_CHAR_PROP_BIT_WRITE_NR);
◆ setWriteProperty()
void BLECharacteristic::setWriteProperty |
( |
bool |
value | ) |
|
Set the Write property value.
- Parameters
-
[in] | value | Set to true if we are to allow writes. |
332 m_properties = (ble_gatt_char_prop_t)(m_properties | BLE_GATT_CHAR_PROP_BIT_WRITE);
334 m_properties = (ble_gatt_char_prop_t)(m_properties & ~BLE_GATT_CHAR_PROP_BIT_WRITE);
◆ toString()
std::string BLECharacteristic::toString |
( |
| ) |
|
Return a string representation of the characteristic.
- Returns
- A string representation of the characteristic.
344 std::stringstream stringstream;
345 stringstream << std::hex << std::setfill(
'0');
346 stringstream <<
"UUID: " << m_bleUUID.
toString() +
", handle: 0x" << std::setw(2) << m_handle;
347 stringstream <<
" " <<
348 ((m_properties & BLE_GATT_CHAR_PROP_BIT_READ) ?
"Read " :
"") <<
349 ((m_properties & BLE_GATT_CHAR_PROP_BIT_WRITE) ?
"Write " :
"") <<
350 ((m_properties & BLE_GATT_CHAR_PROP_BIT_WRITE_NR) ?
"WriteNoResponse " :
"") <<
351 ((m_properties & BLE_GATT_CHAR_PROP_BIT_BROADCAST) ?
"Broadcast " :
"") <<
352 ((m_properties & BLE_GATT_CHAR_PROP_BIT_NOTIFY) ?
"Notify " :
"") <<
353 ((m_properties & BLE_GATT_CHAR_PROP_BIT_INDICATE) ?
"Indicate " :
"");
354 return stringstream.str();
The documentation for this class was generated from the following files:
BLEUUID getUUID()
Get the UUID of the descriptor.
Definition: BLEDescriptor.cpp:87
std::string getValue()
Get the current value.
Definition: BLEValue.cpp:118
std::string toString()
Get a string representation of the UUID.
Definition: BLEUUID.cpp:317
BLEDescriptor * getByUUID(const char *uuid)
Return the descriptor by UUID.
Definition: BLEDescriptorMap.cpp:35
void setBroadcastProperty(bool value)
Set the permission to broadcast. A characteristics has properties associated with it which define wha...
Definition: BLECharacteristic.cpp:178
void setNotifyProperty(bool value)
Set the Notify property value.
Definition: BLECharacteristic.cpp:236
std::string toString()
Return a string representation of the characteristic.
Definition: BLECharacteristic.cpp:343
void setWriteProperty(bool value)
Set the Write property value.
Definition: BLECharacteristic.cpp:329
A model of a BLE UUID.
Definition: BLEUUID.hpp:41
std::string toString()
Return a string representation of the descriptor.
Definition: BLEDescriptor.cpp:159
void setByUUID(const char *uuid, BLEDescriptor *pDescriptor)
Set the descriptor by UUID.
Definition: BLEDescriptorMap.cpp:72
void setValue(uint8_t *data, size_t size)
Set the value of the characteristic.
void setReadProperty(bool value)
Set the Read property value.
Definition: BLECharacteristic.cpp:250
void setIndicateProperty(bool value)
Set the Indicate property value.
Definition: BLECharacteristic.cpp:222
BLECharacteristic(const char *uuid, uint32_t properties=0)
Construct a characteristic.
Definition: BLECharacteristic.cpp:43
void notify(bool is_notification=true)
Send a notify. A notification is a transmission of up to the first 20 bytes of the characteristic val...
uint8_t * getData()
Get a pointer to the data.
Definition: BLEValue.cpp:92
void setWriteNoResponseProperty(bool value)
Set the Write No Response property value.
Definition: BLECharacteristic.cpp:315